Switch statement is used to execute a block of statement based on the switch expression value. An expression must be of type int, short, byte or char. A case value should be a constant literal value and can not be duplicated. Expression value is compared with each case value. If a match found corresponding block of statements will be executed. A break statement is used to terminate the execution of statements. If no case value matches with expression value then default block of statements will be executed. If break statement is not used within case, all matching cases will be executed.
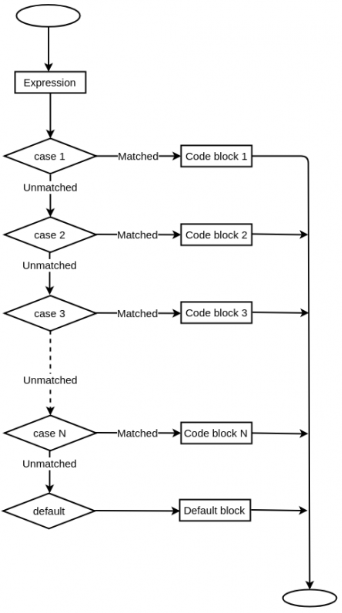
Syntax:
switch(expression){
case value1:
//block of statements
break;
case value2:
//block of statements
break;
...
default:
//block of statements
break;
} |
switch(expression){
case value1:
//block of statements
break;
case value2:
//block of statements
break;
...
default:
//block of statements
break;
}
Program to use switch statement in a Java.
/**
* Program to use switch statement in a Java program.
* @author w3spoint
*/
public class SwitchStatementExample {
static void switchTest(int caseValue){
switch(caseValue) {
case 0:
System.out.println("Case 0.");
break;
case 1:
System.out.println("Case 1.");
break;
case 2:
System.out.println("Case 2.");
break;
default:
System.out.println("Default Case.");
}
}
public static void main(String args[]){
//method call
switchTest(1);
}
} |
/**
* Program to use switch statement in a Java program.
* @author w3spoint
*/
public class SwitchStatementExample {
static void switchTest(int caseValue){
switch(caseValue) {
case 0:
System.out.println("Case 0.");
break;
case 1:
System.out.println("Case 1.");
break;
case 2:
System.out.println("Case 2.");
break;
default:
System.out.println("Default Case.");
}
}
public static void main(String args[]){
//method call
switchTest(1);
}
}
Output:
Download this example.